In this article, we’ll learn how to read files in Python.
In Python, temporary data that is locally used in a module will be stored in a variable. In large volumes of data, a file is used such as text and CSV files and there are methods in Python to read or write data in those files.
After reading this tutorial, you’ll learn: –
- Reading both text and binary files
- The different modes for reading the file
- All methods for reading a text file such as
read()
,readline()
, andreadlines()
- Read text file line by line
- Read and write files at the same time.
Table of contents
Access Modes for Reading a file
To read the contents of a file, we have to open a file in reading mode. Open a file using the built-in function called open()
. In addition to the file name, we need to pass the file mode specifying the purpose of opening the file.
The following are the different modes for reading the file. We will see each one by one.
File Mode | Definition |
---|---|
r | The default mode for opening a file to read the contents of a text file. |
r+ | Open a file for both reading and writing. The file pointer will be placed at the beginning of the file. |
rb | Opens the file for reading a file in binary format. The file pointer will be placed at the beginning of the file. |
w+ | Opens a file for both writing as well as reading. The file pointer will be placed in the beginning of the file. For an existing file, the content will be overwritten. |
a+ | Open the file for both the reading and appending. The pointer will be placed at the end of the file and new content will be written after the existing content. |
Steps for Reading a File in Python
To read a file, Please follow these steps:
- Find the path of a file
We can read a file using both relative path and absolute path. The path is the location of the file on the disk.
An absolute path contains the complete directory list required to locate the file.
A relative path contains the current directory and then the file name. - Open file in Read Mode
To open a file Pass file path and access mode to the
open()
function. The access mode specifies the operation you wanted to perform on the file, such as reading or writing. For example,r
is for reading.
For example,fp= open(r'File_Path', 'r')
- Read content from a file.
Once opened, we can read all the text or content of the file using the
read()
method. You can also use thereadline()
to read file line by line or thereadlines()
to read all lines.
For example,content = fp.read()
- Close file after completing the read operation
We need to make sure that the file will be closed properly after completing the file operation. Use
fp.close()
to close a file.
Example: Read a Text File
The following code shows how to read a text file in Python.
A text file (flatfile) is a kind of computer file that is structured as a sequence of lines of electronic text.
In this example, we are reading all content of a file using the absolute Path.
Consider a file “read_demo.txt.” See the attached file used in the example and an image to show the file’s content for reference.
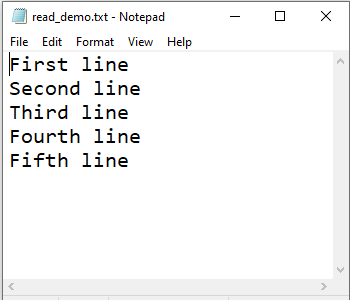
Output
First line Second line Third line Fourth line Fifth line
An absolute path contains the entire path to the file or directory that we need to access. It includes the complete directory list required to locate the file.
For example, E:\PYnative\files_demos\read_demo.txt
is an absolute path to discover the read_demo.txt. All of the information needed to find the file is contained in the path string.
While opening a file for reading its contents we have always ensured that we are providing the correct path. In case the file not present in the provided path we will get FileNotFoundError
.
We can avoid this by wrapping the file opening code in the try-except-finally
block.
Reading a File Using the with
Statement
We can open a file using the with statement along with the open function. The general syntax is as follows.
with open(__file__, accessmode) as f:
Code language: Python (python)
The following are the main advantages of opening a file using ‘with’ statement
- The with statement simplifies exception handling by encapsulating common preparation and cleanup tasks.
- This also ensures that a file is automatically closed after leaving the block.
- As the file is closed automatically it ensures that all the resources that are tied up with the file are released.
Let us see how we can the with statement to read a file.
Output
First line Second line Third line Fourth line Fifth line
File Read Methods
Python provides three different methods to read the file. We don’t have to import any module for that.. Below are the three methods
Method | When to Use? |
---|---|
read() | Returns the entire file content and it accepts the optional size parameter that mentions the bytes to read from the file. |
readline() | The readline() method reads a single line from a file at a time. . Accepts optional size parameter that mentions how many bytes to return from the file. |
readlines() | The readlines () method returns a list of lines from the file. |
readline()
: Read a File Line by Line
Using the readline()
method, we can read a file line by line. by default, this method reads the first line in the file.
For example, If you want to read the first five lines from a text file, run a loop five times, and use the readline()
method in the loop’s body. Using this approach, we can read a specific number of lines.
readline(n)
Code language: Python (python)
Here n
represents the number of bytes to read from the file. This method will read the line and appends a newline character “\n” to the end of the line. While reading a text file this method will return a string.
Output
First line
Reading First N lines From a File Using readline()
We can read the first few set of lines from a file by using the readline()
method. Run a loop fo n times using for loop and range() function, and use the readline()
method in the loop’s body.
Example:
Output
First line Second line Third line
Reading Entire File Using readline()
We can use the readline()
method to read the entire file using the while loop. We need to check whether the pointer has reached the End of the File and then loop through the file line by line.
Output
First line Second line Third line Fourth line Fifth line
Reading First and the Last line using readline()
We can read the first and the last lines of a file using readline()
method. We can get the first line by just calling the readline()
method as this method starts reading from the beginning always and we can use the for
loop to get the last line. This is the better memory-efficient solution as we are not reading the entire file into the memory.
We can understand this better with an example.
Output
First Line Fifth Line
readlines()
: Reading File into List
While we have seen how to extract the entire file contents using the readline()
method, we can achieve the same using the readlines()
method. This method read file line by line into a list.
readlines()
Code language: Python (python)
This method will return the entire file contents. The reading of the contents will start from the beginning of the file till it reaches the EOF (End of File).
This method will internally call the readline()
method and store the contents in a list. The output of this method is a list.
Output
['First line\n', 'Second line\n', 'Third line\n', 'Fourth line\n', 'Fifth line']
As we can see each new line is added as a separate entry in the list with a new line character attached to the end.
Reading first N lines from a file
While the read()
method reads the entire contents of the file we can read only the first few lines by iterating over the file contents.
Let us understand this with an example. Here we are passing the value of N (Number of Lines) from the beginning as 2 and it will return only the first two lines of the file.
Output
['First Line\n', 'Second Line\n']
Reading the last N lines in a file
As we all know the readlines()
method will return the file’s entire content as a list. We can get the last few lines of a file by using the list index and slicing.
Output
['Third Line\n', 'Fourth Line\n', 'Fifth Line']
In the above example we have seen how we can read the last 3 lines in the file by using list slicing.
Reading N Bytes From The File
The read()
method
read([n])
Code language: Python (python)
Here n
represents the number of bytes to read. If nothing is passed, then the entire file contents will be read.
While the read()
method reads the entire file, we can read only a specific number of bytes from the file by passing the parameter ‘n’ to the read()
method.
Let’s see how to read only the first 30 bytes from the file.
Output
First line Second line Third l
Reading and Writing to the same file
Let’s see how to performing multiple operations in a single file. Whenever we try to perform the additional operation after opening a file then it will throw an 'Unsupported Operation'
exception. In case we try to write in a file after opening it for reading operation then it will throw this exception.
Output
UnsupportedOperation: not writable
We can avoid this exception by changing the access mode to r+
. With the access mode set to r+
, the file handle will be placed at the beginning of the file, and then we can read the entire contents. With the write()
method called on the file object, we can overwrite the existing content with new content.
But when we are reading with the r+
the file handle will be in the beginning and we have to make sure that there is a file existing with the name passed. Otherwise, we will get the FileNotFound
exception.
Output
First line
Second line
Third line
Fourth line
Fifth line
Sixth Line
Seventh Line
Code language: Python (python)
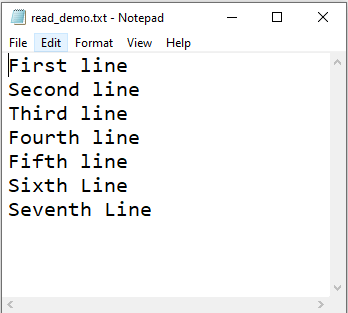
Reading File in Reverse Order
We can read the contents of the file in reverse order by using the readlines()
method and then calling the reversed
() method on the list to get the contents of the list in reverse order. We can then iterate over the contents of the list and print the values.
Output
Fifth Line Fourth Line Third Line Second Line First Line
Reading a Binary file
Binary files are basically the ones with data in the Byte format (0’s and 1’s). This generally doesn’t contain the EOL(End of Line) so it is important to check that condition before reading the contents of the file.
We can open and read the contents of the binary file using the ‘with’ statement as below.
We have seen in this post how the file contents could be read using the different read methods available in Python. We also saw few simple examples to read the contents partially like first few lines or last few lines based on our requirement.
Leave a Reply