Python re.match()
method looks for the regex pattern only at the beginning of the target string and returns match object if match found; otherwise, it will return None.
In this article, You will learn how to match a regex pattern inside the target string using the match()
, search(), and findall() method of a re
module.
The re.match()
method will start matching a regex pattern from the very first character of the text, and if the match found, it will return a re.Match
object. Later we can use the re.Match
object to extract the matching string.
After reading this article you will able to perform the following regex pattern matching operations in Python.
Operation | Meaning |
---|---|
re.match(pattern, str) | Matches pattern only at the beginning of the string |
re.search(pattern, str) | Matches pattern anywhere in the string. Return only first match |
re.search(pattern$, str) | Dollar ($ ) matches pattern at the end of the string. |
re.findall(pattern, str) | Returns all matches to the pattern |
re.findall(^pattern, str, re.M) | Caret (^ ) and re.M flag to match the pattern at the beginning of each new line of a string |
re.fullmatch(pattern, str) | Returns a match object if and only if the entire target string matches the pattern . |
Table of contents
- How to use re.match()
- Match regex pattern at the beginning of the string
- Match regex pattern anywhere in the string
- Match regex at the end of the string
- Match the exact word or string
- Understand the Match object
- Match regex pattern that starts and ends with the given text
- More matching operations
- Regex Search vs. match
- re.fullmatch()
- Why and when to use re.match() and re.fullmatch()
How to use re.match()
Before moving further, let’s see the syntax of re.match()
Syntax of re.match()
re.match(pattern, string, flags=0)
Code language: Python (python)
The regular expression pattern and target string are the mandatory arguments, and flags are optional.
pattern
: The regular expression pattern we want to match at the beginning of the target string. Since we are not defining and compiling this pattern beforehand (like the compile method). The practice is to write the actual pattern using a raw string.string
: The second argument is the variable pointing to the target string (In which we want to look for occurrences of the pattern).flags
: Finally, the third argument is optional and it refers to regex flags by default no flags are applied.
There are many flag values we can use. For example, there.I
is used for performing case-insensitive searching. We can also combine multiple flags using bitwise OR (the|
operator).
Return value
If zero or more characters at the beginning of the string match the regular expression pattern, It returns a corresponding match object instance i.e., re.Match
object. The match object contains the locations at which the match starts and ends and the actual match value.
If it fails to locate the occurrences of the pattern that we want to find or such a pattern doesn’t exist in a target string it will return a None
type

Now, Let’s see how to use re.match()
.
Match regex pattern at the beginning of the string
Now, Let’s see the example to match any four-letter word at the beginning of the string. (Check if the string starts with a given pattern).
Pattern to match: \w{4}
What does this pattern mean?
- The
\w
is a regex special sequence that represents any alphanumeric character meaning letters (uppercase or lowercase), digits, and the underscore character. - Then the 4 inside curly braces say that the character has to occur exactly four times in a row (four consecutive characters).
In simple words, it means to match any four-letter word at the beginning of the following string.
target_string = "Emma is a basketball player who was born on June 17, 1993"
As we can see in the above string Emma is the four-letter word present at the beginning of the target string, so we should get Emma as an output.
As you can see, the match starts at index 0 and ends before index 4. because the re.match()
method always performance pattern matching at the beginning of the target string.
Let’s understand the above example
- I used a raw string to specify the regular expression pattern. As you may already know, the backslash has a special meaning in some cases because it may indicate an escape character or escape sequence to avoid that used raw string.
- Next, we wrote a regex pattern to match any four-letter word.
- Next, we passed this pattern to
match()
method to look for a pattern at the string’s start. - Next, it found a match and returned us the
re.Match
object. - In the end, we used the
group()
method of a Match object to retrieve the exact match value, i.e., Emma.
Match regex pattern anywhere in the string
Let’s assume you want to match any six-letter word inside the following target string
target_string = "Jessa loves Python and pandas"
Code language: Python (python)
If you use a match() method to match any six-letter word inside the string you will get None because it returns a match only if the pattern is located at the beginning of the string. And as we can see the six-letter word is not present at the start.
So to match the regex pattern anywhere in the string you need to use either search()
or findall()
method of a RE module.
Let’s see the demo.
Example to match six-letter word anywhere in the string
Match regex at the end of the string
Sometimes we want to match the pattern at the end of the string. For example, you want to check whether a string is ending with a specific word, number or, character.
Using a dollar ($
) metacharacter we can match the regular expression pattern at the end of the string.
Example to match the four-digit number at the end of the string
Match the exact word or string
In this section, we will see how to write a regex pattern to match an exact word or a substring inside the target string. Let’s see the example to match the word “player” in the target string.
Example:
Understand the Match object
As you know, the match() and search() method returns a re.Match
object if a match found. Let’s see the structure of a re.Match
object.
re.Match object; span=(0, 4), match='Emma'
This re.Match
object contains the following items.
- A span attribute that shows the locations at which the match starts and ends. i.e., is the tuple object contains the start and end index of a successful match.
Save this tuple and use it whenever you want to retrieve a matching string from the target string - Second, A match attribute contains an actual match value that we can retrieve using a
group()
method.
The Match object has several methods and attributes to get the information about the matching string. Let’s see those.
Method | Description |
---|---|
group() | Return the string matched by the regex |
start() | Return the starting position of the match |
end() | Return the ending position of the match |
span() | Return a tuple containing the (start, end) positions of the match. |
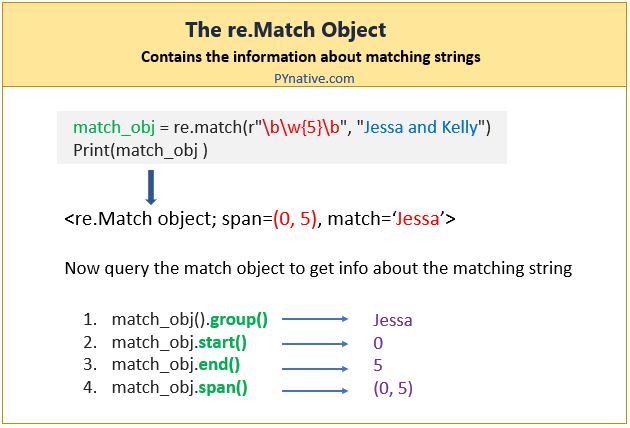
Example to get the information about the matching string
Match regex pattern that starts and ends with the given text
Let’s assume you want to check if a given string starts and ends with a particular text. We can do this using the following two regex metacharacter with re.match()
method.
- Use the caret metacharacter to match at the start
- Use dollar metacharacter to match at the end
Now, let’s check if the given string starts with the letter ‘p’ and ends with the letter ‘t’
Example
More matching operations
In this section, let’s see some common regex matching operations such as
- Match any character
- Match number
- Match digits
- match special characters
Also, read match/capture regex group
Regex Search vs. match
In this section, we will understand the difference between the search() and match() methods. You will also get to know when to use the match and search method while performing regex operations.
Python RE module offers two different methods to perform regex pattern matching.
- The match() checks for a match only at the beginning of the string.
- The search() checks for a match anywhere in the string.
How re.match() works
The match method returns a corresponding match object instance if zero or more characters at the beginning of the string match the regular expression pattern.
In simple words, the re.match
returns a match object only if the pattern is located at the beginning of the string; otherwise, it will return None.
How re.search() works
On the other hand, the search method scans the entire string to look for a pattern and returns only the first match. I.e., As soon as it gets the first match, it stops its execution.
Let’s see the example to understand the difference between search and match. In this example, we will see how to match the regex pattern using the match and search method.
Now, Let’s try to match any2 digit number inside the following target string using search and match method.
Emma is a baseball player who was born on June 17, 1993
As you can see, a two-digit number is not present at the start of a string, So the match() method should return None, and the search method should return the match.
Because the match() method tries to find a match only at the start and search(), try to find a match anywhere in the string.
The behavior of search vs. match with a multiline string
Let’s see example code to understand how the search and match method behaves when a string contains newlines.
We use the re.M
flag with caret (^
) metacharacter to match each regex pattern at each newline’s start. But you must note that even in MULTILINE mode, match() will only match at the beginning of the string and not at the beginning of each line.
On the other hand, the search method scans the entire multi-line string to look for a pattern and returns only the first match
Let’s see the example to understand the difference between search and match when searching inside a multi-line string.
re.fullmatch()
Unlike the match() method, which performs the pattern matching only at the beginning of the string, the re.fullmatch
method returns a match object if and only if the entire target string from the first to the last character matches the regular expression pattern.
If the match performed successfully it will return the entire string as a match value because we always match the entire string in fullmatch
.
For example, you want the target string to have exactly 42 characters in length. Let’s create a regular expression pattern that will check if the target string is 42 characters long.
Pattern to match: .{42}
What does this pattern mean?
This pattern says I want to match a string of 42 characters.
Now let’s have a closer look at the pattern itself. First, you will see the dot in regular expressions syntax.
- The DOT is a special character matching any character, no matter if it’s a letter, digit, whitespace, or a symbol except the newline character, which in Python is a backslash.
- Next, 42 inside the curly braces says that string must be 42 characters long
Now, let’s see the example.
Output:
str1 length: 42 re.Match object; span=(0, 42), match='My name is maximums and my salary is 1000$' Match: My name is maximums and my salary is 1000$
As you can see from the output, we got a match object, meaning the match was performed successfully.
Note: If the string contains one or more newline characters, the match will fail because the special character excludes the new line. Therefore if our target string had had multiple lines or paragraphs, the match would have failed. we cal solve such problems using the flags attribute.
Why and when to use re.match() and re.fullmatch()
- Use
re.match()
method when you want to find the pattern at the beginning of the string (starting with the string’s first character). - If you want to match a full string against a pattern then use
re.fullmatch()
. There.fullmatch
method returns a match object if and only if the entire target string from the first to the last character matches the regular expression pattern.
Previous:
Next:
Leave a Reply